Sometimes you need to wait until some amount of memory becomes free / available to proceed in a bash script.
For such cases MemAvailable in /proc/meminfo can be parsed. The following function checks this against some passed value and also allows to specify a timeout:
Author: Nick Russler
How to Log Cassandra Queries in Java Spring
To enable logging for cassandra queries with Spring Data and the datastax driver you first need to register a QueryLogger instance with your cluster:
1 2 | QueryLogger queryLogger = QueryLogger.builder().build(); cluster.register(queryLogger); |
You also need to specify the log levels for the QueryLogger in your properties:
1 2 3 | logging.level.com.datastax.driver.core.QueryLogger.NORMAL=DEBUG logging.level.com.datastax.driver.core.QueryLogger.SLOW=DEBUG logging.level.com.datastax.driver.core.QueryLogger.ERROR=DEBUG |
While you might not always to turn logging on for NORMAL or even SLOW it can ease debugging a lot.
PHP snippet to filter AirBnB Not available events and fix the end date
I wanted to import the AirBnB calendar via ical into my Google calendar but all the blocked days were annoying. Also the end date was one day too early on all events..
So i hacked a php snippet together which filters the “Not available” events and corrects the date. Then i simply added the php URL to Google calendar and now everything works fine!
If you want to use this snippet just replace the AirBnB URL with your own.
How to fix logrotate cron job error (/etc/cron.daily/logrotate)
After migrating my database to a new server running Ubuntu 14.04.4 LTS i received an email with the following content:
Subject
Cron
test -x /usr/sbin/anacron || ( cd / && run-parts –report /etc/cron.daily )
Body
/etc/cron.daily/logrotate:
error: error running shared postrotate script for ‘/var/log/mysql.log /var/log/mysql/mysql.log /var/log/mysql/mysql-slow.log /var/log/mysql/error.log ‘
run-parts: /etc/cron.daily/logrotate exited with return code 1
The reason why i was getting this error was because i imported my old mysql tables, including the users.
This particular cron job uses a mysql user debian-sys-maint to flush the logs, but i changed the password by importing all of my old tables.
You can fix this by creating the user with the correct password which can be found in the /etc/mysql/debian.cnf file:
Continue reading
How to get random unique integers in a range with Java
If you are able to use Java 8 or up you can make use of the various methods of Random that return an IntStream.
E.g. if you want to generate random distinct numbers from a range [0, 100) you can use:
1 2 3 4 5 | OfInt iterator = ThreadLocalRandom.current().ints(0, 100).distinct().iterator(); for (int i = 0; i < 100; i++) { System.out.println("Next random number: " + iterator.next()); } |
Since our range contains 100 Integers and .distinct() was used we can’t draw more than 100 numbers from the stream!
How to make a notification from a C# Desktop Application in Windows 10
As it seems Microsoft “growls” are called toasts and i like them. I really hope that a lot desktop applications will use this new API to have a single place for all the notifications. Microsoft provides a Quickstart page with a some information and also an example for desktop applications in C++ and C#.
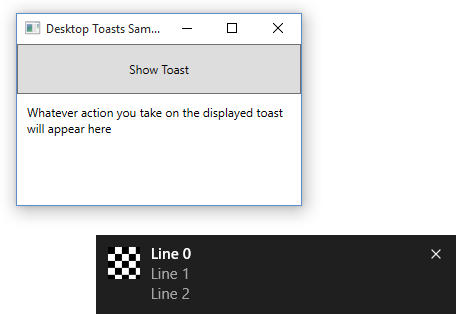
Screenshot of the example Application
Get a list of available Keyboard Models and Layouts on Linux
Most Linux distributions come with the X keyboard extension (XKB) which is part of the X Window System. Fortunately for us the XKB provides a parsable list of all available keyboard models and layouts in /usr/share/X11/xkb/rules/base.xml
. I threw together a Java class that makes use of JAXB to parse this file:
How to convert a String to int / Integer in Java
When you want to convert a String to int or Integer you can use two simple methods from the Integer class.
To convert a String to primitive int use Integer.parseInt(String s)
String a = "1234"; int x = Integer.parseInt(a); |
To convert a String to an instance of Integer use Integer.valueOf(String s)
String a = "1234"; Integer x = Integer.valueOf(a); |
How to compare Strings in Java
A common mistake Java novices do is using the == operator to compare Strings. This does almost certainly lead to unexpected and unwanted behaviour. There is a simple solution to this problem and a not so simple explanation why it is such a common mistake.
The simple solution is to use String.equals instead of the == operator.
So when you want to know if two String objects hold the same value instead of
String a = "Test"; String b = "Test"; if (a == b) { // Do something } |
just use this:
String a = "Test"; String b = "Test"; if (a.equals(b)) { // Do something } |
But look out for null Strings, == handles null Strings nicely but when you call
String a = null; String b = "Test"; if (a.equals(b)) { // Do something } |
it will result in a NullPointerException!
Self-update Firefox Addon programatically
Sometimes you can’t wait for Firefox’s built in update mechanism to update you addons. Say you want to let your addon check for a new version on every startup of Firefox and then trigger the installtion of the new version. This can be done with a short Snippet:
This code surely could use some error handling and such, so feel free to throw your enhancements at me!